mirror of
https://github.com/reactos/reactos.git
synced 2024-07-09 06:05:11 +00:00
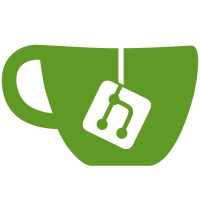
* sumatrapdf - vendor import * everything compiles (libjpeg, poppler, fitz, sumatrapdf) * does NOT link (remove the comment tags in the parent directory.rbuild file (rosapps dir) to build it) svn path=/trunk/; revision=29295
71 lines
1.3 KiB
C
71 lines
1.3 KiB
C
/* Written by Krzysztof Kowalczyk (http://blog.kowalczyk.info)
|
|
The author disclaims copyright to this source code. */
|
|
#include "strlist_util.h"
|
|
#include "str_util.h"
|
|
|
|
int StrList_Len(StrList **root)
|
|
{
|
|
int len = 0;
|
|
StrList * cur;
|
|
assert(root);
|
|
if (!root)
|
|
return 0;
|
|
cur = *root;
|
|
while (cur) {
|
|
++len;
|
|
cur = cur->next;
|
|
}
|
|
return len;
|
|
}
|
|
|
|
BOOL StrList_InsertAndOwn(StrList **root, char *txt)
|
|
{
|
|
StrList * el;
|
|
assert(root && txt);
|
|
if (!root || !txt)
|
|
return FALSE;
|
|
|
|
el = (StrList*)malloc(sizeof(StrList));
|
|
if (!el)
|
|
return FALSE;
|
|
el->str = txt;
|
|
el->next = *root;
|
|
*root = el;
|
|
return TRUE;
|
|
}
|
|
|
|
BOOL StrList_Insert(StrList **root, char *txt)
|
|
{
|
|
char *txtDup;
|
|
|
|
assert(root && txt);
|
|
if (!root || !txt)
|
|
return FALSE;
|
|
txtDup = str_dup(txt);
|
|
if (!txtDup)
|
|
return FALSE;
|
|
|
|
if (!StrList_InsertAndOwn(root, txtDup)) {
|
|
free((void*)txtDup);
|
|
return FALSE;
|
|
}
|
|
return TRUE;
|
|
}
|
|
|
|
void StrList_Destroy(StrList **root)
|
|
{
|
|
StrList * cur;
|
|
StrList * next;
|
|
|
|
if (!root)
|
|
return;
|
|
cur = *root;
|
|
while (cur) {
|
|
next = cur->next;
|
|
free((void*)cur->str);
|
|
free((void*)cur);
|
|
cur = next;
|
|
}
|
|
*root = NULL;
|
|
}
|