mirror of
https://github.com/reactos/reactos.git
synced 2024-06-29 01:12:06 +00:00
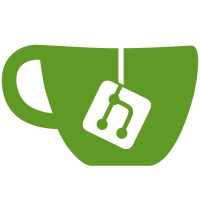
Fix vertical alignment and Aspect penalty; improve font penalty system; fixes for Wine tests. This is an update of #706. See CORE-11536 for more details. The display of the following programs is fixed: - Total Commander 8.52 setup: font displayed too large - CORE-11620. - Effective File Search 6.8.1 german localization text rendering issues - CORE-14378. - Font garbage in register splash screen in Foxit Reader 7.1.5 - CORE-9767. - Calipers-1 is not displayed correctly - CORE-14302. - Some MSI-installers draw their dialogs too large (example: Click-N-Type Virtual Keyboard 3.03.0412) - CORE-13161. - Irfanview 4.50: font in zoom combobox displayed too large - CORE-14396. - Rufus: The window and controls are displayed larger than necessary - CORE-14461.
182 lines
4.6 KiB
C
182 lines
4.6 KiB
C
/*
|
|
* ReactOS kernel
|
|
* Copyright (C) 1998, 1999, 2000, 2001 ReactOS Team
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS kernel
|
|
* PURPOSE: GDI Internal Objects
|
|
* FILE: win32ss/gdi/eng/engobjects.h
|
|
* PROGRAMER: Jason Filby
|
|
* REVISION HISTORY:
|
|
* 21/8/1999: Created
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
/* Structure of internal gdi objects that win32k manages for ddi engine:
|
|
|---------------------------------|
|
|
| Public part |
|
|
| accessed from engine |
|
|
|---------------------------------|
|
|
| Private part |
|
|
| managed by gdi |
|
|
|_________________________________|
|
|
|
|
---------------------------------------------------------------------------*/
|
|
|
|
typedef struct _RWNDOBJ {
|
|
PVOID pvConsumer;
|
|
RECTL rclClient;
|
|
SURFOBJ *psoOwner;
|
|
} RWNDOBJ;
|
|
|
|
/* EXtended CLip and Window Region Objects */
|
|
#ifdef __cplusplus
|
|
typedef struct _XCLIPOBJ : _CLIPOBJ, _RWNDOBJ
|
|
{
|
|
#else
|
|
typedef struct _XCLIPOBJ
|
|
{
|
|
CLIPOBJ;
|
|
RWNDOBJ;
|
|
#endif
|
|
struct _REGION *pClipRgn; /* prgnRao_ or (prgnVis_ if (prgnRao_ == z)) */
|
|
//
|
|
RECTL rclClipRgn;
|
|
//PVOID pscanClipRgn; /* Ptr to regions rect buffer based on iDirection. */
|
|
RECTL* Rects;
|
|
DWORD cScan;
|
|
DWORD reserved;
|
|
ULONG EnumPos;
|
|
LONG lscnSize;
|
|
ULONG EnumMax;
|
|
ULONG iDirection;
|
|
ULONG iType;
|
|
DWORD reserved1;
|
|
LONG lUpDown;
|
|
DWORD reserved2;
|
|
BOOL bAll;
|
|
//
|
|
DWORD RectCount; /* count/mode based on # of rect in regions scan. */
|
|
PVOID pDDA; /* Pointer to a large drawing structure. */
|
|
} XCLIPOBJ, *PXCLIPOBJ;
|
|
|
|
/*
|
|
EngCreateClip allocates XCLIPOBJ and REGION, pco->co.pClipRgn = &pco->ro.
|
|
{
|
|
XCLIPOBJ co;
|
|
REGION ro;
|
|
}
|
|
*/
|
|
|
|
extern XCLIPOBJ gxcoTrivial;
|
|
|
|
#ifdef __cplusplus
|
|
typedef struct _EWNDOBJ : _XCLIPOBJ
|
|
{
|
|
#else
|
|
typedef struct _EWNDOBJ
|
|
{
|
|
XCLIPOBJ;
|
|
#endif
|
|
/* Extended WNDOBJ part */
|
|
HWND Hwnd;
|
|
WNDOBJCHANGEPROC ChangeProc;
|
|
FLONG Flags;
|
|
int PixelFormat;
|
|
} EWNDOBJ, *PEWNDOBJ;
|
|
|
|
|
|
/*ei What is this for? */
|
|
typedef struct _DRVFUNCTIONSGDI {
|
|
HDEV hdev;
|
|
DRVFN Functions[INDEX_LAST];
|
|
} DRVFUNCTIONSGDI;
|
|
|
|
typedef struct _FLOATGDI {
|
|
ULONG Dummy;
|
|
} FLOATGDI;
|
|
|
|
typedef struct _SHARED_MEM {
|
|
PVOID Buffer;
|
|
ULONG BufferSize;
|
|
BOOL IsMapping;
|
|
LONG RefCount;
|
|
} SHARED_MEM, *PSHARED_MEM;
|
|
|
|
typedef struct _SHARED_FACE_CACHE {
|
|
UINT OutlineRequiredSize;
|
|
UNICODE_STRING FontFamily;
|
|
UNICODE_STRING FullName;
|
|
} SHARED_FACE_CACHE, *PSHARED_FACE_CACHE;
|
|
|
|
typedef struct _SHARED_FACE {
|
|
FT_Face Face;
|
|
LONG RefCount;
|
|
PSHARED_MEM Memory;
|
|
SHARED_FACE_CACHE EnglishUS;
|
|
SHARED_FACE_CACHE UserLanguage;
|
|
} SHARED_FACE, *PSHARED_FACE;
|
|
|
|
typedef struct _FONTGDI {
|
|
FONTOBJ FontObj;
|
|
ULONG iUnique;
|
|
FLONG flType;
|
|
|
|
DHPDEV dhpdev;
|
|
PSHARED_FACE SharedFace;
|
|
|
|
LONG lMaxNegA;
|
|
LONG lMaxNegC;
|
|
LONG lMinWidthD;
|
|
|
|
LPWSTR Filename;
|
|
BYTE RequestUnderline;
|
|
BYTE RequestStrikeOut;
|
|
BYTE RequestItalic;
|
|
LONG RequestWeight;
|
|
BYTE OriginalItalic;
|
|
LONG OriginalWeight;
|
|
BYTE CharSet;
|
|
|
|
/* Precomputed font metrics (supplements FreeType metrics) */
|
|
LONG tmHeight;
|
|
LONG tmAscent;
|
|
LONG tmDescent;
|
|
LONG tmInternalLeading;
|
|
LONG EmHeight;
|
|
LONG Magic;
|
|
} FONTGDI, *PFONTGDI;
|
|
|
|
/* The initialized 'Magic' value in FONTGDI */
|
|
#define FONTGDI_MAGIC 0x20110311
|
|
|
|
typedef struct _PATHGDI {
|
|
PATHOBJ PathObj;
|
|
} PATHGDI;
|
|
|
|
typedef struct _XFORMGDI {
|
|
ULONG Dummy;
|
|
/* XFORMOBJ has no public members */
|
|
} XFORMGDI;
|
|
|
|
/* As the *OBJ structures are located at the beginning of the *GDI structures
|
|
we can simply typecast the pointer */
|
|
#define ObjToGDI(ClipObj, Type) (Type##GDI *)(ClipObj)
|
|
#define GDIToObj(ClipGDI, Type) (Type##OBJ *)(ClipGDI)
|