mirror of
https://github.com/reactos/reactos.git
synced 2025-01-05 22:12:46 +00:00
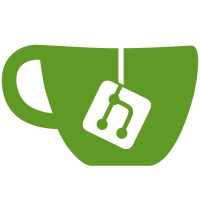
- Implement the save/load handler for this feature - Check the "Use Click Sound" item state accordingly depending if it's enabled or disabled - Use PlaySoundW() to play the wave sound file from resource - Add the WAV sound click file. The work is made thanks to Midori Mizuno
176 lines
5.1 KiB
C
176 lines
5.1 KiB
C
/*
|
|
* PROJECT: ReactOS On-Screen Keyboard
|
|
* LICENSE: GPL-2.0+ (https://spdx.org/licenses/GPL-2.0+)
|
|
* PURPOSE: Settings file for warning dialog on startup
|
|
* COPYRIGHT: Copyright 2018 Bișoc George (fraizeraust99 at gmail dot com)
|
|
*/
|
|
|
|
/* INCLUDES *******************************************************************/
|
|
|
|
#include "osk.h"
|
|
#include "settings.h"
|
|
|
|
/* FUNCTIONS *******************************************************************/
|
|
|
|
BOOL LoadDataFromRegistry()
|
|
{
|
|
HKEY hKey;
|
|
LONG lResult;
|
|
DWORD dwShowWarningData, dwLayout, dwSoundOnClick;
|
|
DWORD cbData = sizeof(DWORD);
|
|
|
|
/* Set the structure members to TRUE (and the bSoundClick member to FALSE) */
|
|
Globals.bShowWarning = TRUE;
|
|
Globals.bIsEnhancedKeyboard = TRUE;
|
|
Globals.bSoundClick = FALSE;
|
|
|
|
/* Open the key, so that we can query it */
|
|
lResult = RegOpenKeyExW(HKEY_CURRENT_USER,
|
|
L"Software\\Microsoft\\osk",
|
|
0,
|
|
KEY_READ,
|
|
&hKey);
|
|
|
|
if (lResult != ERROR_SUCCESS)
|
|
{
|
|
/* Bail out and return FALSE if we fail */
|
|
return FALSE;
|
|
}
|
|
|
|
/* Query the key */
|
|
lResult = RegQueryValueExW(hKey,
|
|
L"ShowWarning",
|
|
0,
|
|
0,
|
|
(BYTE *)&dwShowWarningData,
|
|
&cbData);
|
|
|
|
if (lResult != ERROR_SUCCESS)
|
|
{
|
|
/* Bail out and return FALSE if we fail */
|
|
RegCloseKey(hKey);
|
|
return FALSE;
|
|
}
|
|
|
|
/* Load the data value (it can be either FALSE or TRUE depending on the data itself) */
|
|
Globals.bShowWarning = (dwShowWarningData != 0);
|
|
|
|
/* Query the key */
|
|
lResult = RegQueryValueExW(hKey,
|
|
L"IsEnhancedKeyboard",
|
|
0,
|
|
0,
|
|
(BYTE *)&dwLayout,
|
|
&cbData);
|
|
|
|
if (lResult != ERROR_SUCCESS)
|
|
{
|
|
/* Bail out and return FALSE if we fail */
|
|
RegCloseKey(hKey);
|
|
return FALSE;
|
|
}
|
|
|
|
/* Load the dialog layout value */
|
|
Globals.bIsEnhancedKeyboard = (dwLayout != 0);
|
|
|
|
/* Query the key */
|
|
lResult = RegQueryValueExW(hKey,
|
|
L"OnSoundClick",
|
|
0,
|
|
0,
|
|
(BYTE *)&dwSoundOnClick,
|
|
&cbData);
|
|
|
|
if (lResult != ERROR_SUCCESS)
|
|
{
|
|
/* Bail out and return FALSE if we fail */
|
|
RegCloseKey(hKey);
|
|
return FALSE;
|
|
}
|
|
|
|
/* Load the sound on click value event */
|
|
Globals.bSoundClick = (dwSoundOnClick != 0);
|
|
|
|
/* If we're here then we succeed, close the key and return TRUE */
|
|
RegCloseKey(hKey);
|
|
return TRUE;
|
|
}
|
|
|
|
BOOL SaveDataToRegistry()
|
|
{
|
|
HKEY hKey;
|
|
LONG lResult;
|
|
DWORD dwShowWarningData, dwLayout, dwSoundOnClick;
|
|
|
|
/* If no key has been made, create one */
|
|
lResult = RegCreateKeyExW(HKEY_CURRENT_USER,
|
|
L"Software\\Microsoft\\osk",
|
|
0,
|
|
NULL,
|
|
0,
|
|
KEY_WRITE,
|
|
NULL,
|
|
&hKey,
|
|
NULL);
|
|
|
|
if (lResult != ERROR_SUCCESS)
|
|
{
|
|
/* Bail out and return FALSE if we fail */
|
|
return FALSE;
|
|
}
|
|
|
|
/* The data value of the subkey will be appended to the warning dialog switch */
|
|
dwShowWarningData = Globals.bShowWarning;
|
|
|
|
lResult = RegSetValueExW(hKey,
|
|
L"ShowWarning",
|
|
0,
|
|
REG_DWORD,
|
|
(BYTE *)&dwShowWarningData,
|
|
sizeof(dwShowWarningData));
|
|
|
|
if (lResult != ERROR_SUCCESS)
|
|
{
|
|
/* Bail out and return FALSE if we fail */
|
|
RegCloseKey(hKey);
|
|
return FALSE;
|
|
}
|
|
|
|
/* The value will be appended to the layout dialog */
|
|
dwLayout = Globals.bIsEnhancedKeyboard;
|
|
|
|
lResult = RegSetValueExW(hKey,
|
|
L"IsEnhancedKeyboard",
|
|
0,
|
|
REG_DWORD,
|
|
(BYTE *)&dwLayout,
|
|
sizeof(dwLayout));
|
|
|
|
if (lResult != ERROR_SUCCESS)
|
|
{
|
|
/* Bail out and return FALSE if we fail */
|
|
RegCloseKey(hKey);
|
|
return FALSE;
|
|
}
|
|
|
|
/* The value will be appended to the sound on click event */
|
|
dwSoundOnClick = Globals.bSoundClick;
|
|
|
|
lResult = RegSetValueExW(hKey,
|
|
L"OnSoundClick",
|
|
0,
|
|
REG_DWORD,
|
|
(BYTE *)&dwSoundOnClick,
|
|
sizeof(dwSoundOnClick));
|
|
|
|
if (lResult != ERROR_SUCCESS)
|
|
{
|
|
/* Bail out and return FALSE if we fail */
|
|
RegCloseKey(hKey);
|
|
return FALSE;
|
|
}
|
|
|
|
/* If we're here then we succeed, close the key and return TRUE */
|
|
RegCloseKey(hKey);
|
|
return TRUE;
|
|
}
|