mirror of
https://github.com/reactos/reactos.git
synced 2024-07-05 12:15:46 +00:00
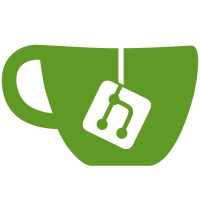
Two issues are addressed: CORE-2189: missing undo feature Works the same way as solitaire in windows xp: - only 1 action can be undone; - the player gets -2 points in standard score mode; - the undo action resets when the player clicks on a row stack to turn the top card. CORE-11148: invisible cards This happens in 3-card mode, when only 1 card left in the deck. The fix for this is to modify the pile stack to contain all the face-up cards. It was actually already in the code somewhere else, so I turned it into a separate function.
77 lines
2.6 KiB
C++
77 lines
2.6 KiB
C++
#include "solitaire.h"
|
|
|
|
CardRegion *pDeck;
|
|
CardRegion *pPile;
|
|
CardRegion *pSuitStack[4];
|
|
CardRegion *pRowStack[NUM_ROW_STACKS];
|
|
|
|
HBITMAP hbmBitmap;
|
|
HDC hdcBitmap;
|
|
int yRowStackCardOffset;
|
|
|
|
void CreateSol()
|
|
{
|
|
int i;
|
|
|
|
// hbmBitmap = (HBITMAP)LoadImage(0,"test.bmp",IMAGE_BITMAP,0,0,LR_LOADFROMFILE);
|
|
// SolWnd.SetBackImage(hbmBitmap);
|
|
|
|
activepile.Clear();
|
|
|
|
// Compute the value for yRowStackCardOffset based on the height of the card, so the card number isn't hidden on larger cards
|
|
yRowStackCardOffset = (int)(__cardheight / 6.7);
|
|
|
|
pDeck = SolWnd.CreateRegion(DECK_ID, true, X_BORDER, Y_BORDERWITHFRAME, 2, 1);
|
|
pDeck->SetEmptyImage(CS_EI_CIRC);
|
|
pDeck->SetThreedCount(6);
|
|
pDeck->SetDragRule(CS_DRAG_NONE, 0);
|
|
pDeck->SetDropRule(CS_DROP_NONE, 0);
|
|
pDeck->SetClickProc(DeckClickProc);
|
|
pDeck->SetDblClickProc(DeckClickProc);
|
|
pDeck->SetFaceDirection(CS_FACE_DOWN, 0);
|
|
|
|
pPile = SolWnd.CreateRegion(PILE_ID, true, X_BORDER + __cardwidth + X_PILE_BORDER, Y_BORDERWITHFRAME, CS_DEFXOFF, 1);
|
|
pPile->SetEmptyImage(CS_EI_NONE);
|
|
pPile->SetDragRule(CS_DRAG_TOP, 0);
|
|
pPile->SetDropRule(CS_DROP_NONE, 0);
|
|
pPile->SetDblClickProc(PileDblClickProc);
|
|
pPile->SetRemoveCardProc(PileRemoveProc);
|
|
pPile->SetClickProc(PileClickProc);
|
|
|
|
//
|
|
// Create the suit stacks
|
|
//
|
|
for(i = 0; i < 4; i++)
|
|
{
|
|
pSuitStack[i] = SolWnd.CreateRegion(SUIT_ID+i, true, 0, Y_BORDERWITHFRAME, 0, 0);
|
|
pSuitStack[i]->SetEmptyImage(CS_EI_SUNK);
|
|
pSuitStack[i]->SetPlacement(CS_XJUST_CENTER, 0, i * (__cardwidth + X_SUITSTACK_BORDER) , 0);
|
|
|
|
pSuitStack[i]->SetDropRule(CS_DROP_CALLBACK, SuitStackDropProc);
|
|
pSuitStack[i]->SetDragRule(CS_DRAG_TOP);
|
|
pSuitStack[i]->SetClickProc(SuitStackClickProc);
|
|
|
|
pSuitStack[i]->SetAddCardProc(SuitStackAddProc);
|
|
}
|
|
|
|
//
|
|
// Create the row stacks
|
|
//
|
|
for(i = 0; i < NUM_ROW_STACKS; i++)
|
|
{
|
|
pRowStack[i] = SolWnd.CreateRegion(ROW_ID+i, true, 0, Y_BORDERWITHFRAME + __cardheight + Y_ROWSTACK_BORDER, 0, yRowStackCardOffset);
|
|
pRowStack[i]->SetEmptyImage(CS_EI_SUNK);
|
|
pRowStack[i]->SetFaceDirection(CS_FACE_DOWNUP, i);
|
|
|
|
pRowStack[i]->SetPlacement(CS_XJUST_CENTER, 0,
|
|
(i - NUM_ROW_STACKS/2) * (__cardwidth + X_ROWSTACK_BORDER), 0);
|
|
|
|
pRowStack[i]->SetEmptyImage(CS_EI_NONE);
|
|
|
|
pRowStack[i]->SetDragRule(CS_DRAG_CALLBACK, RowStackDragProc);
|
|
pRowStack[i]->SetDropRule(CS_DROP_CALLBACK, RowStackDropProc);
|
|
pRowStack[i]->SetClickProc(RowStackClickProc);
|
|
pRowStack[i]->SetDblClickProc(RowStackDblClickProc);
|
|
}
|
|
}
|