mirror of
https://github.com/reactos/reactos.git
synced 2025-02-23 17:05:46 +00:00
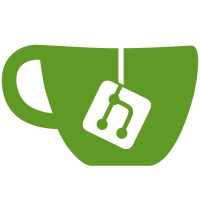
Fixed an error in the accepting code (in rostcp.c line 576). tcp_accepted was being called for the wrong pcb. Instead of the pcb belonging to the listening socket it was being called for the pcb belonging to the newly created connection socket. In order to fix this I added an extra field to the tcp_pcb structure to hold a reference to the listening socket. This is a crude method and it will be replaced by something more elegant. Right now however there's a slight nondeterminism regarding the connection. One it's established the message either gets through to the other side or not, randomly. This could be due to a race condition of some sorts. Also another problem is that the server side brings down the system when closing. svn path=/branches/GSoC_2011/TcpIpDriver/; revision=51889
80 lines
2.2 KiB
C
80 lines
2.2 KiB
C
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS TCP/IP protocol driver
|
|
* FILE: include/debug.h
|
|
* PURPOSE: Debugging support macros
|
|
* DEFINES: DBG - Enable debug output
|
|
* NASSERT - Disable assertions
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#define MIN_TRACE ((1 << DPFLTR_WARNING_LEVEL))
|
|
#define MID_TRACE ((1 << DPFLTR_WARNING_LEVEL) | (1 << DPFLTR_TRACE_LEVEL))
|
|
#define MAX_TRACE ((1 << DPFLTR_WARNING_LEVEL) | (1 << DPFLTR_TRACE_LEVEL) | (1 << DPFLTR_INFO_LEVEL))
|
|
|
|
#define DEBUG_CHECK 0x00000100
|
|
#define DEBUG_MEMORY 0x00000200
|
|
#define DEBUG_PBUFFER 0x00000400
|
|
#define DEBUG_IRP 0x00000800
|
|
#define DEBUG_TCPIF 0x00001000
|
|
#define DEBUG_ADDRFILE 0x00002000
|
|
#define DEBUG_DATALINK 0x00004000
|
|
#define DEBUG_ARP 0x00008000
|
|
#define DEBUG_IP 0x00010000
|
|
#define DEBUG_UDP 0x00020000
|
|
#define DEBUG_TCP 0x00040000
|
|
#define DEBUG_ICMP 0x00080000
|
|
#define DEBUG_ROUTER 0x00100000
|
|
#define DEBUG_RCACHE 0x00200000
|
|
#define DEBUG_NCACHE 0x00400000
|
|
#define DEBUG_CPOINT 0x00800000
|
|
#define DEBUG_LOCK 0x01000000
|
|
#define DEBUG_INFO 0x02000000
|
|
#define DEBUG_ULTRA 0x7FFFFFFF
|
|
|
|
#define DBG 1
|
|
|
|
#if DBG
|
|
|
|
#define REMOVE_PARENS(...) __VA_ARGS__
|
|
#define TI_DbgPrint(_t_, _x_) \
|
|
DbgPrintEx(DPFLTR_TCPIP_ID, (_t_) | DPFLTR_MASK, "(%s:%d) ", __FILE__, __LINE__), \
|
|
DbgPrintEx(DPFLTR_TCPIP_ID, (_t_) | DPFLTR_MASK, REMOVE_PARENS _x_)
|
|
//DbgPrint(REMOVE_PARENS _x_)
|
|
#else /* DBG */
|
|
|
|
#define TI_DbgPrint(_t_, _x_)
|
|
|
|
#endif /* DBG */
|
|
|
|
|
|
#define assert(x) ASSERT(x)
|
|
#define assert_irql(x) ASSERT_IRQL(x)
|
|
|
|
|
|
#ifdef _MSC_VER
|
|
|
|
#define UNIMPLEMENTED \
|
|
TI_DbgPrint(MIN_TRACE, ("The function at %s:%d is unimplemented, \
|
|
but come back another day.\n", __FILE__, __LINE__));
|
|
|
|
#else /* _MSC_VER */
|
|
|
|
#define UNIMPLEMENTED \
|
|
TI_DbgPrint(MIN_TRACE, ("(%s:%d)(%s) is unimplemented, \
|
|
but come back another day.\n", __FILE__, __LINE__, __FUNCTION__));
|
|
|
|
#endif /* _MSC_VER */
|
|
|
|
|
|
#define CHECKPOINT \
|
|
do { TI_DbgPrint(DEBUG_CHECK, ("(%s:%d)\n", __FILE__, __LINE__)); } while(0);
|
|
|
|
#define CP CHECKPOINT
|
|
|
|
#define ASSERT_KM_POINTER(_x) \
|
|
ASSERT(((PVOID)_x) != (PVOID)0xcccccccc); \
|
|
ASSERT(((PVOID)_x) >= (PVOID)0x80000000);
|
|
|
|
/* EOF */
|