mirror of
https://github.com/reactos/reactos.git
synced 2024-07-04 19:54:58 +00:00
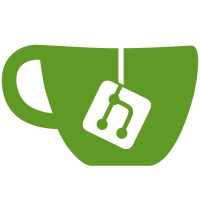
The server-side CsrSrvIdentifyAlertableThread and CsrSrvSetPriorityClass functions are completely removed in Win2k3+, and are since stubbed by CsrSrvUnusedFunction instead. They however were present up to Windows XP, albeit with an extremely minimal implementation. The corresponding client-side CsrIdentifyAlertableThread and CsrSetPriorityClass now become just stubs that either trivially succeed or fail, respectively. See https://www.geoffchappell.com/studies/windows/win32/csrsrv/api/srvinit/apidispatch.htm for more information. - Fix typo "al*T*ertable" --> "alertable". - Remove ROS-specific CSRSS_IDENTIFY_ALERTABLE_THREAD that was deprecated since ages (at least before 2005)!
101 lines
2.5 KiB
C
101 lines
2.5 KiB
C
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS kernel
|
|
* FILE: dll/ntdll/csr/api.c
|
|
* PURPOSE: CSR APIs exported through NTDLL
|
|
* PROGRAMMER: Alex Ionescu (alex@relsoft.net)
|
|
*/
|
|
|
|
/* INCLUDES *******************************************************************/
|
|
|
|
#include <ntdll.h>
|
|
|
|
#define NDEBUG
|
|
#include <debug.h>
|
|
|
|
/* GLOBALS ********************************************************************/
|
|
|
|
extern HANDLE CsrApiPort;
|
|
|
|
/* FUNCTIONS ******************************************************************/
|
|
|
|
/*
|
|
* @implemented
|
|
*/
|
|
NTSTATUS
|
|
NTAPI
|
|
CsrNewThread(VOID)
|
|
{
|
|
/* Register the termination port to CSR's */
|
|
return NtRegisterThreadTerminatePort(CsrApiPort);
|
|
}
|
|
|
|
/*
|
|
* @implemented
|
|
*/
|
|
NTSTATUS
|
|
NTAPI
|
|
CsrIdentifyAlertableThread(VOID)
|
|
{
|
|
#if (NTDDI_VERSION < NTDDI_WS03)
|
|
NTSTATUS Status;
|
|
CSR_API_MESSAGE ApiMessage;
|
|
PCSR_IDENTIFY_ALERTABLE_THREAD IdentifyAlertableThread;
|
|
|
|
/* Set up the data for CSR */
|
|
IdentifyAlertableThread = &ApiMessage.Data.IdentifyAlertableThread;
|
|
IdentifyAlertableThread->Cid = NtCurrentTeb()->ClientId;
|
|
|
|
/* Call it */
|
|
Status = CsrClientCallServer(&ApiMessage,
|
|
NULL,
|
|
CSR_CREATE_API_NUMBER(CSRSRV_SERVERDLL_INDEX, CsrpIdentifyAlertableThread),
|
|
sizeof(*IdentifyAlertableThread));
|
|
|
|
/* Return to caller */
|
|
return Status;
|
|
#else
|
|
/* Deprecated */
|
|
return STATUS_SUCCESS;
|
|
#endif
|
|
}
|
|
|
|
/*
|
|
* @implemented
|
|
*/
|
|
NTSTATUS
|
|
NTAPI
|
|
CsrSetPriorityClass(IN HANDLE Process,
|
|
IN OUT PULONG PriorityClass)
|
|
{
|
|
#if (NTDDI_VERSION < NTDDI_WS03)
|
|
NTSTATUS Status;
|
|
CSR_API_MESSAGE ApiMessage;
|
|
PCSR_SET_PRIORITY_CLASS SetPriorityClass = &ApiMessage.Data.SetPriorityClass;
|
|
|
|
/* Set up the data for CSR */
|
|
SetPriorityClass->hProcess = Process;
|
|
SetPriorityClass->PriorityClass = *PriorityClass;
|
|
|
|
/* Call it */
|
|
Status = CsrClientCallServer(&ApiMessage,
|
|
NULL,
|
|
CSR_CREATE_API_NUMBER(CSRSRV_SERVERDLL_INDEX, CsrpSetPriorityClass),
|
|
sizeof(*SetPriorityClass));
|
|
|
|
/* Return what we got, if requested */
|
|
if (*PriorityClass) *PriorityClass = SetPriorityClass->PriorityClass;
|
|
|
|
/* Return to caller */
|
|
return Status;
|
|
#else
|
|
UNREFERENCED_PARAMETER(Process);
|
|
UNREFERENCED_PARAMETER(PriorityClass);
|
|
|
|
/* Deprecated */
|
|
return STATUS_INVALID_PARAMETER;
|
|
#endif
|
|
}
|
|
|
|
/* EOF */
|