mirror of
https://github.com/reactos/reactos.git
synced 2024-06-27 08:21:31 +00:00
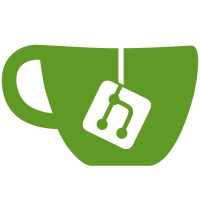
Addendum to608032bd
and835c3023
. The IRQL is actually raised by KeFreezeExecution() and lowered by KeThawExecution(), always to HIGH_IRQL on MP systems, or if necessary on UP. These functions are called respectively by KdEnterDebugger() and KdExitDebugger().
85 lines
1.9 KiB
C
85 lines
1.9 KiB
C
/*
|
|
* PROJECT: ReactOS Kernel
|
|
* LICENSE: GPL - See COPYING in the top level directory
|
|
* FILE: ntoskrnl/ke/freeze.c
|
|
* PURPOSE: Routines for freezing and unfreezing processors for
|
|
* kernel debugger synchronization.
|
|
* PROGRAMMERS: Alex Ionescu (alex.ionescu@reactos.org)
|
|
*/
|
|
|
|
/* INCLUDES *******************************************************************/
|
|
|
|
#include <ntoskrnl.h>
|
|
#define NDEBUG
|
|
#include <debug.h>
|
|
|
|
/* GLOBALS ********************************************************************/
|
|
|
|
/* Freeze data */
|
|
KIRQL KiOldIrql;
|
|
ULONG KiFreezeFlag;
|
|
|
|
/* FUNCTIONS ******************************************************************/
|
|
|
|
BOOLEAN
|
|
NTAPI
|
|
KeFreezeExecution(IN PKTRAP_FRAME TrapFrame,
|
|
IN PKEXCEPTION_FRAME ExceptionFrame)
|
|
{
|
|
BOOLEAN Enable;
|
|
KIRQL OldIrql;
|
|
|
|
#ifndef CONFIG_SMP
|
|
UNREFERENCED_PARAMETER(TrapFrame);
|
|
UNREFERENCED_PARAMETER(ExceptionFrame);
|
|
#endif
|
|
|
|
/* Disable interrupts, get previous state and set the freeze flag */
|
|
Enable = KeDisableInterrupts();
|
|
KiFreezeFlag = 4;
|
|
|
|
#ifndef CONFIG_SMP
|
|
/* Raise IRQL if we have to */
|
|
OldIrql = KeGetCurrentIrql();
|
|
if (OldIrql < DISPATCH_LEVEL)
|
|
OldIrql = KeRaiseIrqlToDpcLevel();
|
|
#else
|
|
/* Raise IRQL to HIGH_LEVEL */
|
|
KeRaiseIrql(HIGH_LEVEL, &OldIrql);
|
|
#endif
|
|
|
|
#ifdef CONFIG_SMP
|
|
// TODO: Add SMP support.
|
|
#endif
|
|
|
|
/* Save the old IRQL to be restored on unfreeze */
|
|
KiOldIrql = OldIrql;
|
|
|
|
/* Return whether interrupts were enabled */
|
|
return Enable;
|
|
}
|
|
|
|
VOID
|
|
NTAPI
|
|
KeThawExecution(IN BOOLEAN Enable)
|
|
{
|
|
#ifdef CONFIG_SMP
|
|
// TODO: Add SMP support.
|
|
#endif
|
|
|
|
/* Clear the freeze flag */
|
|
KiFreezeFlag = 0;
|
|
|
|
/* Cleanup CPU caches */
|
|
KeFlushCurrentTb();
|
|
|
|
/* Restore the old IRQL */
|
|
#ifndef CONFIG_SMP
|
|
if (KiOldIrql < DISPATCH_LEVEL)
|
|
#endif
|
|
KeLowerIrql(KiOldIrql);
|
|
|
|
/* Re-enable interrupts */
|
|
KeRestoreInterrupts(Enable);
|
|
}
|