mirror of
https://github.com/reactos/reactos.git
synced 2024-06-01 02:01:57 +00:00
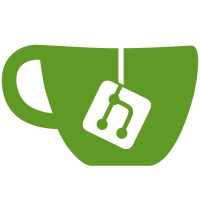
Refactoring and reduce binary size. JIRA issue: CORE-19268 - Add cicero static library in sdk/lib/cicero folder. - Delete sdk/include/reactos/cicero folder. - Adapt the dependencies to these changes. - Make ctfmon, msutb, and msctf modules UNICODE.
51 lines
1,004 B
C++
51 lines
1,004 B
C++
/*
|
|
* PROJECT: ReactOS Cicero
|
|
* LICENSE: LGPL-2.1-or-later (https://spdx.org/licenses/LGPL-2.1-or-later)
|
|
* PURPOSE: Cicero mutex handling
|
|
* COPYRIGHT: Copyright 2023 Katayama Hirofumi MZ <katayama.hirofumi.mz@gmail.com>
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include "cicbase.h"
|
|
|
|
class CicMutex
|
|
{
|
|
HANDLE m_hMutex;
|
|
BOOL m_bInit;
|
|
|
|
public:
|
|
CicMutex() : m_hMutex(NULL), m_bInit(FALSE)
|
|
{
|
|
}
|
|
~CicMutex()
|
|
{
|
|
Uninit();
|
|
}
|
|
|
|
void Init(LPSECURITY_ATTRIBUTES lpSA, LPCTSTR pszMutexName)
|
|
{
|
|
m_hMutex = ::CreateMutex(lpSA, FALSE, pszMutexName);
|
|
m_bInit = TRUE;
|
|
}
|
|
void Uninit()
|
|
{
|
|
if (m_hMutex)
|
|
{
|
|
::CloseHandle(m_hMutex);
|
|
m_hMutex = NULL;
|
|
}
|
|
m_bInit = FALSE;
|
|
}
|
|
|
|
BOOL Enter()
|
|
{
|
|
DWORD dwWait = ::WaitForSingleObject(m_hMutex, 5000);
|
|
return (dwWait == WAIT_OBJECT_0) || (dwWait == WAIT_ABANDONED);
|
|
}
|
|
void Leave()
|
|
{
|
|
::ReleaseMutex(m_hMutex);
|
|
}
|
|
};
|